Programming with the Arduino IDE pt II - Challenges
1. Use nested loops to fill a 3D array.
Answer
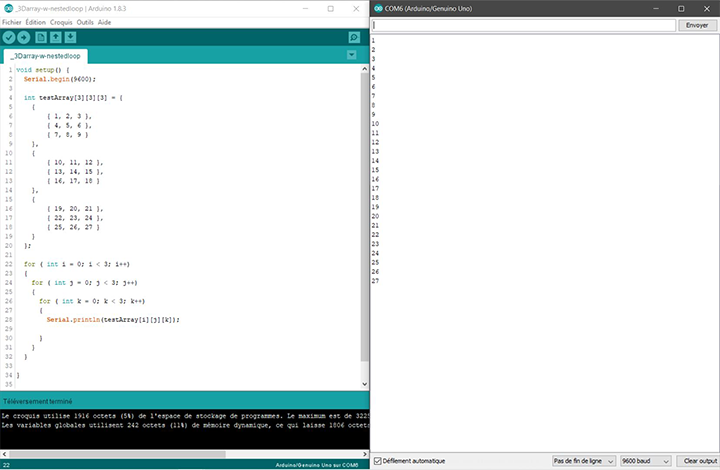
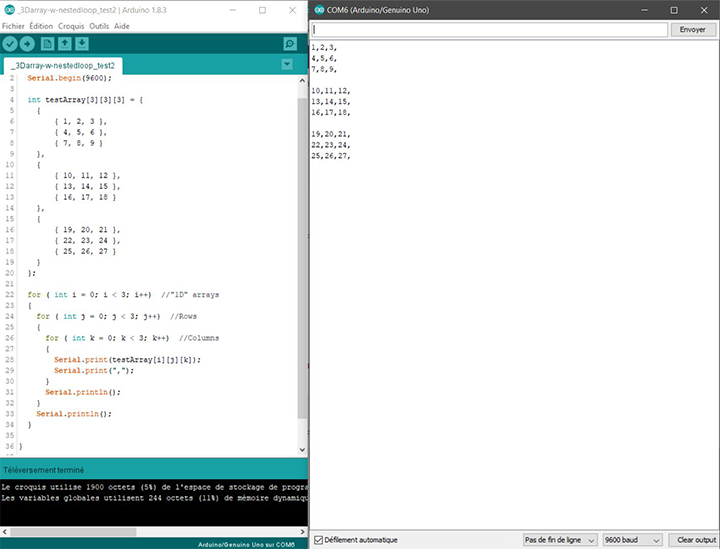
Code
void setup() {
Serial.begin(9600);
int testArray[3][3][3] = { //initialize the 3d array
{
{ 1, 2, 3 },
{ 4, 5, 6 },
{ 7, 8, 9 }
},
{
{ 10, 11, 12 },
{ 13, 14, 15 },
{ 16, 17, 18 }
},
{
{ 19, 20, 21 },
{ 22, 23, 24 },
{ 25, 26, 27 }
}
};
for ( int i = 0; i < 3; i++) //"1D" arrays
{
for ( int j = 0; j < 3; j++) //Rows
{
for ( int k = 0; k < 3; k++) //Columns
{
Serial.print(testArray[i][j][k]); //print columns, rows, and arrays values
Serial.print(","); //print a comma
}
Serial.println(); //new line for new row
}
Serial.println(); //new line for new array
}
}
void loop() {
}
2. Write a code to produce this result in the serial monitor:
5
44
333
2222
11111
Answer
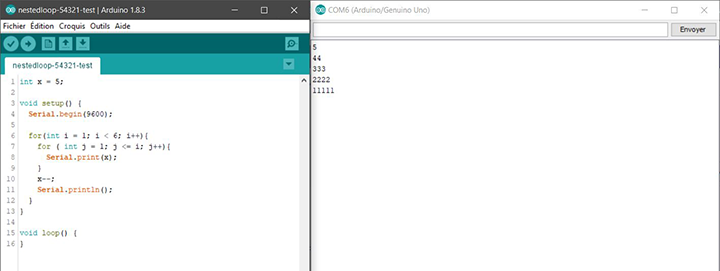
Code
int x = 5; //declaring a global value x
void setup() {
Serial.begin(9600);
for(int i = 1; i < 6; i++){ //incrementing lines
for ( int j = 1; j <= i; j++){ //incrementing columns
Serial.print(x); //printing x value
}
x--; //decrementing x value
Serial.println(); //new line
}
}
void loop() {
}
3. Write a code to produce this result in the serial monitor:
*
**
***
****
*****
****
***
**
*
Answer
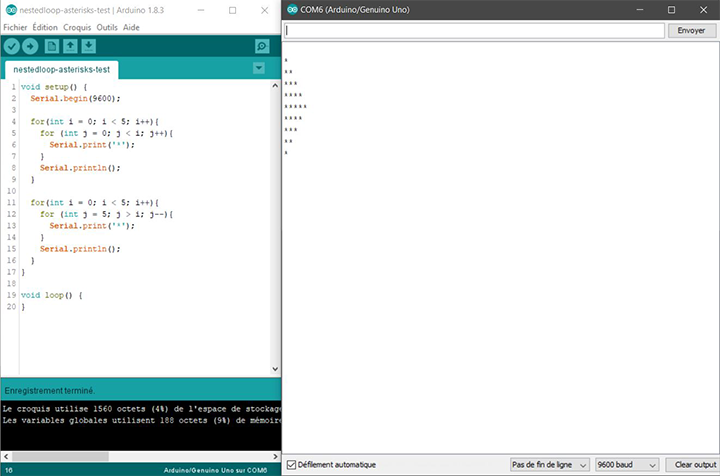
Code
void setup() {
Serial.begin(9600);
for(int i = 0; i < 5; i++){ //incrementing lines
for (int j = 0; j < i; j++){ //incrementing columns
Serial.print('*'); //print "*" when column incrementing
}
Serial.println(); //print a new line when line incrementing
}
for(int i = 0; i < 5; i++){ //incrementing lines
for (int j = 5; j > i; j--){ //decrementing columns
Serial.print('*'); //print "*" when column decrementingcrementing
}
Serial.println(); //print a new line when line incrementing
}
}
void loop() {
}
4. Using a nested loop for each sentence in a paragraph, count the number of times “the” appears in a given text (saved in a String, for instance).
https://www.arduino.cc/reference/en/language/variables/data-types/string/
https://www.arduino.cc/en/Tutorial/BuiltInExamples/StringSubstring